1. Introduction to Spring Framework and Transaction Management
1.1 Overview of Spring Framework
The Spring Framework is an essential tool in the modern software development landscape, renowned for its robustness, flexibility, and comprehensive suite of features that streamline the development process. Originally developed to counter the complexities of early Java Enterprise Edition (JEE) solutions, Spring has evolved into a go-to framework for creating high-performing, scalable applications.
At its core, Spring is built around the principle of dependency injection (DI) and aspect-oriented programming (AOP), allowing developers to create loosely coupled, manageable components. These components can be easily integrated, tested, and maintained, making Spring particularly suitable for enterprise-level applications.
Key features of the Spring Framework include:
- Inversion of Control (IoC) Container: Central to Spring, this feature manages the lifecycle and configuration of application objects.
- Spring MVC: A model-view-controller framework that simplifies the development of web applications.
- Data Access Framework: Provides a consistent approach to data access, whether it’s JDBC, Hibernate, JPA, or other ORM technologies.
- Spring Boot: A convention-over-configuration solution that accelerates application development by simplifying Spring setup.
- Spring Security: Offers comprehensive security services for enterprise applications.
1.2 Importance of Transaction Management in Software Development
Transaction management is a critical component in software development, particularly for applications dealing with data storage and retrieval. In simple terms, a transaction is a sequence of operations performed as a single, atomic unit. This concept is vital in ensuring data integrity and consistency, especially in systems where multiple concurrent operations occur.
Effective transaction management means that either all operations in a transaction are successfully completed, or none are, maintaining the database’s integrity. This is particularly crucial in applications like banking systems, e-commerce platforms, and any other system where data consistency is paramount.
1.3 Role of @Transactional in Spring
In the realm of Spring Framework, the @Transactional annotation plays a pivotal role in simplifying transaction management. By abstracting the underlying transaction handling, it allows developers to focus on business logic rather than the intricacies of transaction management.
@Transactional offers several benefits:
- Declarative Transaction Management: Instead of writing boilerplate code to handle transactions, developers can use this annotation to declaratively manage transactions.
- Consistency and Safety: It ensures that the data remains consistent and safe throughout the transaction lifecycle.
- Reduced Boilerplate Code: Reduces the amount of boilerplate code required for transaction management, thereby improving code readability and maintainability.
- Flexibility and Customization: Allows customization of transactional behavior, such as propagation, isolation levels, timeout settings, and more.
In the following sections, we will delve deeper into how @Transactional works, its implementation, and best practices to leverage its full potential in Spring-based applications. We’ll explore the technical aspects that make @Transactional a powerful annotation for managing transactions, ensuring data integrity, and streamlining the development process in complex enterprise applications.
2. Understanding Transactions
2.1 Definition and Importance of Transactions
In software development, a transaction typically refers to a series of operations or actions that are treated as a single unit of work. These operations must either all succeed or, if any of them fail, the entire transaction should fail and the system should revert to its previous state. This all-or-nothing approach is crucial for maintaining data integrity and consistency, especially in systems where data is constantly being read from and written to a database.
Transactions are particularly important in applications that require a high level of data reliability, such as financial systems, e-commerce platforms, and customer data management systems. In these environments, the accuracy and consistency of data are paramount, and transaction management plays a critical role in ensuring these requirements are met.
2.2 ACID Properties of Transactions
The concept of transactions in computing is often explained through the ACID properties, which stand for Atomicity, Consistency, Isolation, and Durability:
- Atomicity: This property ensures that all operations within a transaction are completed successfully. If any operation fails, the entire transaction is rolled back, meaning no operation within the failed transaction is applied to the database.
- Consistency: Transactions must leave the database in a consistent state. This means that every transaction should start with the database in a consistent state and end with the database in a consistent state, even if the transaction is rolled back.
- Isolation: This property ensures that transactions are protected from the interference of other concurrent transactions. It maintains data accuracy by ensuring that concurrent transactions cannot access data in an inconsistent state.
- Durability: Once a transaction has been committed, it will remain so, even in the event of a system failure. This property ensures that the results of the operations are permanently stored in the database.
2.3 Challenges in Transaction Management
Managing transactions effectively can be challenging due to several factors:
- Complexity of State Management: Ensuring that the database remains in a consistent state throughout a transaction can be complex, especially when dealing with large volumes of data and multiple concurrent transactions.
- Performance Overhead: Implementing transaction management, particularly in distributed systems, can introduce performance overhead. This is due to the additional processing required to ensure ACID properties.
- Deadlocks and Concurrency Issues: Deadlocks can occur when two or more transactions are waiting for each other to release locks. Handling such scenarios and other concurrency issues is a key challenge in transaction management.
- Scalability: As applications grow, managing transactions across a distributed database architecture becomes increasingly challenging.
Understanding these challenges is crucial for developers, as it helps in choosing the right transaction management strategy and in writing more efficient and reliable code. In the next section, we will explore how the @Transactional annotation in Spring addresses these challenges and simplifies transaction management in Java applications.
3. Deep Dive into @Transactional Annotation
3.1 Basic Usage of @Transactional
The @Transactional annotation in Spring is a declarative way of managing transactions in your Java applications. When a method annotated with @Transactional is executed, Spring Framework automatically starts a new transaction. This simplification removes the need for manual transaction handling (like explicitly starting or committing a transaction) and lets developers focus on business logic.
A typical usage of @Transactional might look like this:
package at.ciit.spring.example1;
import at.ciit.spring.CustomException;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class MyService {
@Transactional
public void myTransactionalMethod() {
// Business logic that requires transactional support
}
}
In this example, myTransactionalMethod will be executed within a transactional context provided by Spring.
3.2 How Spring Implements Transaction Management
Spring Framework’s transaction management is implemented using a combination of AOP (Aspect-Oriented Programming) and proxying. When a class is annotated with @Transactional or contains methods with this annotation, Spring dynamically creates a proxy that wraps the class. This proxy is responsible for managing the transaction lifecycle (start, commit, rollback) based on the method’s execution outcome.
Here’s a simplified view of the process:
- Proxy Creation: When the application starts, Spring scans for @Transactional annotations and creates proxies for the affected beans.
- Transaction Handling: When a transactional method is invoked, the proxy intercepts the call and starts a new transaction (or joins an existing one, depending on the propagation settings).
- Commit or Rollback: If the method completes successfully, the transaction is committed. If an exception is thrown, the transaction is rolled back.
3.3 Behind the Scenes: Proxy Mechanism and AOP
The proxy mechanism is central to how @Transactional works. Spring uses either JDK dynamic proxies (when interfaces are available) or CGLIB proxies (for class-based proxying) to create these transactional proxies. The choice between JDK and CGLIB proxies can be influenced by various factors, including whether the target object implements an interface.
AOP plays a crucial role here, as it allows the separation of transaction management from business logic. The @Transactional annotation is, in essence, an aspect that Spring applies to the target method. This aspect manages the transaction lifecycle transparently, ensuring that the method is executed within the bounds of a transaction.
This section sets the stage for understanding the various attributes and configurations available with @Transactional, which will be explored in the subsequent sections. These attributes allow for fine-tuning transactional behavior to suit different requirements and scenarios, making @Transactional a powerful and flexible tool in the Spring ecosystem.
4. Core Concepts of Transaction Management in Spring
4.1 Propagation Behavior of Transactions
One of the key aspects of @Transactional is the control of transaction boundaries through propagation behavior. Spring provides several options for propagation, which determine how transactions are handled relative to each other. The most commonly used propagation behaviors include:
- REQUIRED (default): Supports the current transaction; creates a new one if none exists.
- REQUIRES_NEW: Creates a new transaction, suspending the current one if it exists.
- SUPPORTS: Runs within a transaction if one is already present; otherwise, runs non-transactionally.
- NOT_SUPPORTED: Executes non-transactionally, suspending any current transaction.
- MANDATORY: Supports the current transaction; throws an exception if no current transaction exists.
- NEVER: Ensures the method is not run within a transaction; throws an exception if a transaction exists.
- NESTED: Executes within a nested transaction if a current transaction exists; otherwise, behaves like REQUIRED.
Understanding these behaviors is crucial for correctly managing transaction demarcation, especially in complex applications with multiple transactional methods interacting with each other.
4.2 Isolation Levels in Transactions
Isolation levels define how data accessed by one transaction is isolated from other transactions. In Spring, the @Transactional annotation allows specifying the isolation level, which is critical for preventing concurrency-related issues like dirty reads, non-repeatable reads, and phantom reads. The standard isolation levels include:
- DEFAULT: Uses the default isolation level of the underlying datastore.
- READ_UNCOMMITTED: Allows dirty reads; one transaction may see uncommitted changes made by another.
- READ_COMMITTED: Prevents dirty reads; data read is committed at the point of reading.
- REPEATABLE_READ: Ensures repeatable reads; data read cannot change during the transaction.
- SERIALIZABLE: The highest level; complete isolation from other transactions.
Choosing the appropriate isolation level depends on the specific requirements of the application and its tolerance for concurrency side-effects.
4.3 Rollback Rules and Exception Handling
Spring provides a flexible way to define rollback behavior in @Transactional. By default, a transaction will roll back on runtime, unchecked exceptions (like RuntimeException) but not on checked exceptions. However, this behavior can be customized using the rollbackFor and noRollbackFor attributes of the @Transactional annotation.
For example:
package at.ciit.spring.example2;
import at.ciit.spring.CustomException;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class MyService {
@Transactional(rollbackFor = {CustomException.class})
public void myMethod() {
// Business logic
}
}
This configuration specifies that the transaction should roll back for CustomException.
Understanding and correctly configuring rollback rules is vital for maintaining data integrity and handling exceptions effectively in a transactional context.
5. Advanced Features of @Transactional
5.1 Programmatically Managing Transactions
While the @Transactional annotation provides a declarative way of managing transactions, Spring also allows for programmatic transaction management. This can be particularly useful in scenarios where you need more control over the transaction, such as in complex business logic that requires dynamic transaction management.
Programmatic transaction management in Spring is typically achieved through the PlatformTransactionManager interface. Here’s a simple example:
package at.ciit.spring.example3;
import org.springframework.transaction.PlatformTransactionManager;
import org.springframework.transaction.TransactionStatus;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.transaction.support.DefaultTransactionDefinition;
@Transactional
public class MyService {
private final PlatformTransactionManager transactionManager;
public MyService(PlatformTransactionManager transactionManager) {
this.transactionManager = transactionManager;
}
public void myMethod() {
DefaultTransactionDefinition def = new DefaultTransactionDefinition();
TransactionStatus status = transactionManager.getTransaction(def);
try {
// Business logic
transactionManager.commit(status);
} catch (Exception ex) {
transactionManager.rollback(status);
throw ex;
}
}
}
In this example, the PlatformTransactionManager is used to manually start, commit, and roll back transactions.
5.2 Transaction Synchronization and Event Handling
Spring provides the ability to synchronize business logic with transaction events, such as on transaction completion or rollback. This feature is valuable for performing certain operations only after the transaction has been committed or rolled back.
You can register a TransactionSynchronization with Spring’s TransactionSynchronizationManager, which provides callback methods like beforeCommit, afterCommit, beforeCompletion, afterCompletion, etc. These methods can be overridden to execute custom logic at specific points in the transaction lifecycle.
5.3 Custom Transaction Strategies with @Transactional
The flexibility of the @Transactional annotation extends to allowing the development of custom transaction strategies. This is achieved by combining various attributes of the annotation, such as propagation, isolation, timeout, readOnly, and the rules for exception handling (rollbackFor and noRollbackFor).
For instance, you can create a transactional method that:
- Starts a new transaction if none exists (propagation=REQUIRES_NEW).
- Has a custom timeout (timeout=5), meaning the transaction will be rolled back if it takes more than 5 seconds.
- Is read-only (readOnly=true), which can optimize performance for read-only operations.
package at.ciit.spring.example4;
import org.springframework.transaction.annotation.Propagation;
import org.springframework.transaction.annotation.Transactional;
@Transactional
public class MyService {
@Transactional(propagation = Propagation.REQUIRES_NEW, timeout = 5, readOnly = true)
public void myReadOnlyOperation() {
// Read-only business logic
}
}
These advanced features of @Transactional enable developers to create sophisticated and highly customizable transaction management strategies. This flexibility is essential for addressing the diverse needs of complex enterprise applications.
In the next section, we will discuss best practices and common pitfalls to be aware of when using @Transactional in Spring applications.
6. Best Practices and Common Pitfalls
6.1 Proper Usage of @Transactional in Application Layers
One of the most critical best practices in using @Transactional is understanding where it should be applied within the application layers. Commonly, it is most effective at the service layer rather than the data access layer (DAO) or the controller layer. This approach ensures that the transactional boundaries are around business logic, thereby providing better control over transactions and making the system more robust.
- Service Layer: Ideal for @Transactional as it typically encapsulates business logic and calls multiple DAO methods, which should be part of the same transaction.
- Data Access Layer (DAO): Avoid using @Transactional here, as it can lead to multiple transactions within a single business process.
- Controller Layer: Generally not recommended, as controllers should not be aware of transaction management.
6.2 Performance Considerations and Optimizations
Transaction management can have a significant impact on application performance. It’s important to consider the following:
- Avoid Long Transactions: Long-running transactions can hold database locks for extended periods, impacting performance. Keep transactions as short as possible.
- Read-Only Transactions: Mark transactions as read-only whenever possible. This can optimize database performance and resource utilization.
- Lazy Loading: Be cautious with lazy loading within transactions. Accessing lazy-loaded data outside of the transactional context can lead to issues like LazyInitializationException.
6.3 Troubleshooting Common Issues
When working with @Transactional, developers may encounter certain issues. Here are some common pitfalls and how to address them:
- Transaction Not Starting: Ensure that the method with @Transactional is being called from outside its own class. Transactions won’t start if the method is called internally due to the way Spring AOP works.
- Unexpected Rollbacks: Be aware of the default rollback behavior. Spring rolls back on unchecked exceptions but not on checked ones. Customize this behavior with the rollbackFor attribute if necessary.
- Proxying Issues: Remember that Spring uses proxies for transaction management. Final classes and methods can’t be proxied using Spring’s default AOP proxying mechanism.
- Isolation Level Conflicts: Understand the impact of different isolation levels on your application, especially in terms of performance and concurrency behavior.
Adhering to these best practices and being aware of common pitfalls will greatly enhance the effectiveness of @Transactional in your Spring applications. The next sections will cover case studies and real-world scenarios, providing practical insights into applying these concepts effectively.
7. Case Studies and Real-World Scenarios
7.1 Integrating @Transactional with Various Database Systems
Different database systems, like MySQL, PostgreSQL, Oracle, and NoSQL databases, can have varying behaviors and requirements for transaction management. How @Transactional is leveraged can significantly impact its effectiveness in different database environments.
Case Study: Transitioning from Oracle to MySQL
In this scenario, a financial application initially developed for Oracle is migrated to MySQL. The development team must adjust the transaction management strategies due to differences in default isolation levels and locking behaviors between these databases. For instance, Oracle’s default level of READ_COMMITTED might need to be explicitly set in MySQL to avoid issues like phantom reads.
Best Practices:
- Test transactional behavior thoroughly when switching databases.
- Adjust isolation levels and transactional settings according to the database’s characteristics.
7.2 Transaction Management in Distributed Systems
Managing transactions across microservices or distributed architectures introduces additional complexity. This is because operations may span multiple databases or external systems, requiring coordinated transactions.
Case Study: Implementing Distributed Transactions in Microservices
A retail company uses microservices for different aspects of its business – orders, inventory, and shipping. Implementing a transaction that spans these services requires a strategy like two-phase commit or employing a distributed transaction management framework.
Best Practices:
- Avoid distributed transactions if possible by designing each service to be self-contained.
- If unavoidable, use patterns like Saga to manage distributed transactions.
7.3 Lessons from Real-World Applications
Real-world applications often provide valuable insights into the practical challenges and solutions in transaction management.
Case Study: E-Commerce Platform Scalability
An e-commerce platform experiences high transaction volumes during peak sales periods. The platform needs to handle concurrent transactions efficiently while maintaining data integrity.
Best Practices:
- Implement appropriate isolation levels to balance data consistency and performance.
- Use read replicas for read-only transactions to reduce the load on the primary database.
7.4 Handling Complex Transactions with Spring
Complex transactions, such as those involving conditional logic or multiple data sources, require careful planning and implementation.
Case Study: Financial Reporting System
A financial system needs to generate reports by aggregating data from multiple sources. This process involves complex transactions where partial failure in data fetching should not halt the entire reporting process.
Best Practices:
- Use REQUIRES_NEW or NESTED propagation for subtransactions that should be independent.
- Handle exceptions strategically to ensure partial success of the overall transaction.
8. Conclusion
8.1 Summarizing the Role and Impact of @Transactional
The @Transactional annotation in the Spring Framework stands out as a pivotal element in simplifying transaction management in Java applications. Throughout this article, we have explored the depths of @Transactional, from its basic usage and internal workings to advanced features and best practices. Its ability to manage transaction boundaries declaratively makes it an invaluable tool for developers, enabling them to focus on business logic rather than the intricacies of transaction handling.
8.2 Key Takeaways
- Ease of Use: @Transactional provides a straightforward, declarative approach to handle transactions, drastically reducing boilerplate code.
- Flexibility: Through various attributes like propagation, isolation levels, and custom rollback rules, @Transactional offers flexibility to cater to diverse transaction management needs.
- Enhanced Reliability and Consistency: It ensures that data integrity and consistency are maintained across transactions, which is crucial for the robustness of enterprise applications.
- Performance Considerations: Understanding the nuances of @Transactional can lead to better performance optimizations and more efficient application design.
8.3 Future Trends in Transaction Management
As technology evolves, so does the landscape of transaction management. We are likely to see advancements in areas like:
- Distributed Transaction Management: With the rise of microservices and distributed architectures, new patterns and tools for managing distributed transactions will emerge.
- Integration with Reactive Programming: As reactive programming gains popularity, transaction management in reactive environments will become more relevant.
- Enhanced Support for NoSQL Databases: As NoSQL databases become more prevalent, we can expect improved transaction support and optimizations in this area.
8.4 Final Thoughts
@Transactional in Spring represents a powerful abstraction for transaction management, blending simplicity with flexibility. The continued evolution of Spring and its transaction management capabilities is poised to keep pace with the changing demands of modern application development.
As we conclude, it’s evident that a deep understanding of @Transactional and transaction management principles is essential for building reliable, high-performing, and maintainable Java applications. By leveraging the insights and best practices discussed in this article, developers can harness the full potential of @Transactional in their Spring applications.
9. References and Further Reading
In order to deepen your understanding of @Transactional in Spring and related topics, the following resources are highly recommended. These materials range from official documentation to insightful books and articles, providing both foundational knowledge and advanced insights into transaction management with Spring.
9.1 Official Documentation
- Spring Framework Documentation:
9.2 Books
- „Spring in Action“ by Craig Walls:
- A highly regarded book in the Spring community, offering practical examples and in-depth explanations.
- Covers various aspects of Spring, including transaction management.
- „Pro Spring 6: An In-Depth Guide to the Spring Framework“
- Provides a comprehensive exploration of Spring 5, including detailed coverage of transaction management.
10. Further Examples
10.1 A Service & 2 Repositories in one transaction
@Transactional
, ensuring that both repository calls are part of the same transaction.
package at.ciit.spring.example10_1;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.dao.DataAccessException;
@Service
public class MySpringService {
private final MyFirstRepository firstRepository;
private final MySecondRepository secondRepository;
@Autowired
public MySpringService(MyFirstRepository firstRepository, MySecondRepository secondRepository) {
this.firstRepository = firstRepository;
this.secondRepository = secondRepository;
}
@Transactional
public void updateData(DataObject1 dataObject1, DataObject2 dataObject2) {
try {
// First repository call
firstRepository.save(dataObject1);
// Second repository call
secondRepository.save(dataObject2);
} catch (DataAccessException ex) {
// Exception handling logic
// The transaction will automatically roll back in case of any DataAccessException
throw ex;
}
}
}
package at.ciit.spring.example10_1;
import org.springframework.data.jpa.repository.JpaRepository;
// Example repository interfaces
public interface MyFirstRepository extends JpaRepository {
// Custom methods for DataObject1
}
package at.ciit.spring.example10_1;
import org.springframework.data.jpa.repository.JpaRepository;
public interface MySecondRepository extends JpaRepository {
// Custom methods for DataObject2
}
In this example:
- The
MySpringService
class is marked as a Spring service using@Service
. - The
updateData
method, annotated with@Transactional
, ensures that both operations onfirstRepository
andsecondRepository
are within the same transaction boundary. - If any exception, particularly
DataAccessException
, occurs during the execution of either repository method, the transaction will automatically roll back, maintaining data integrity. - The
MyFirstRepository
andMySecondRepository
are typical Spring Data repository interfaces extendingJpaRepository
, tailored forDataObject1
andDataObject2
entities respectively.
This code demonstrates how Spring’s @Transactional
annotation can be used to manage transactions spanning multiple repository calls in a cohesive and streamlined manner.
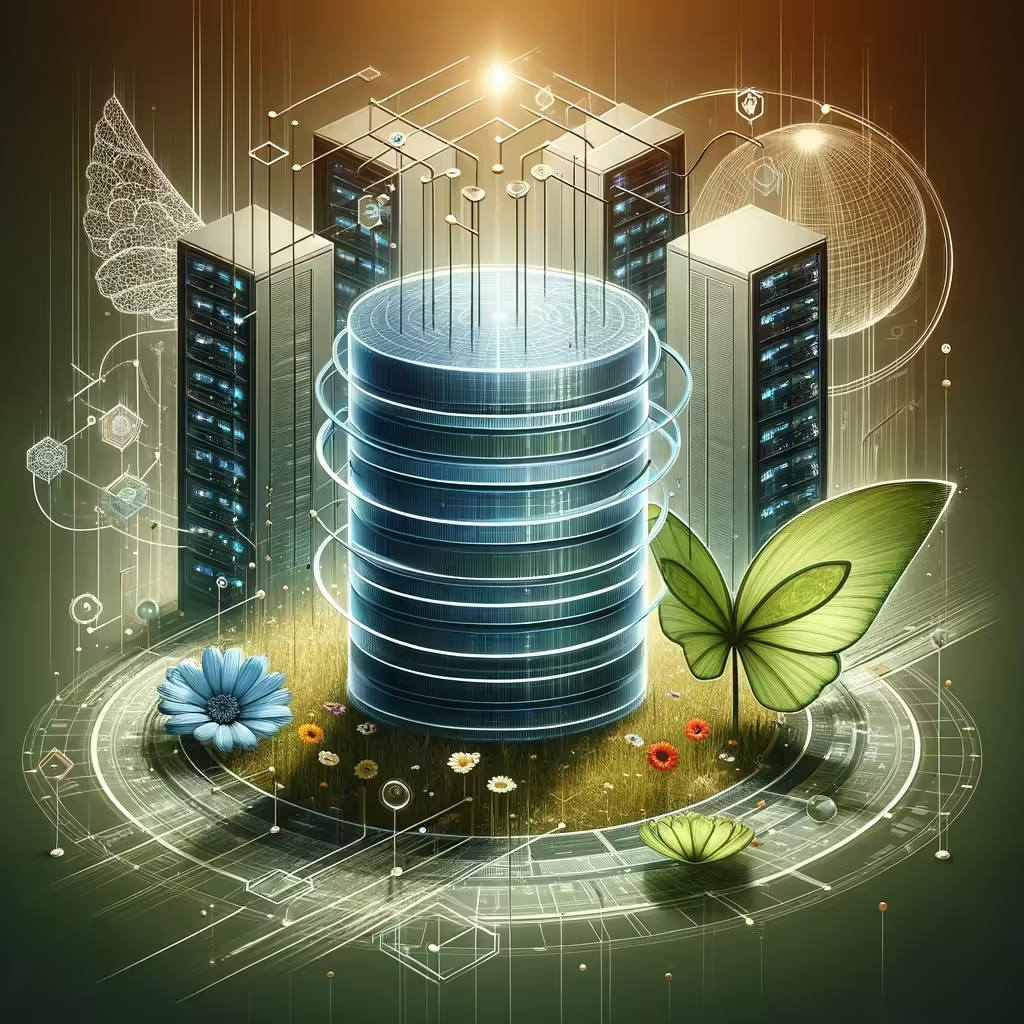